Using Repository Records & Google Sheets
Ironclad's Contract Repository enables companies to store, search, and report on executed contracts and structured contract properties. However, the data stored in these contracts may be valuable across many different applications. In this guide, we will show how to export contract information into another application, such as a Data Lake or Data Warehouse.
Note: This guide and any software contained in it should be used at your own risk by individuals qualified to evaluate its effectiveness. IT IS PROVIDED “AS IS”, WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL IRONCLAD BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH YOUR USE OF THIS GUIDE OR ANY SOFTWARE IT CONTAINS.
Your use of the Ironclad API must comply with Ironclad’s API Terms of Use (available at https://legal.ironcladapp.com/api-terms-of-use) and the terms of the Enterprise Services Agreement (or equivalent) entered into between you and Ironclad.
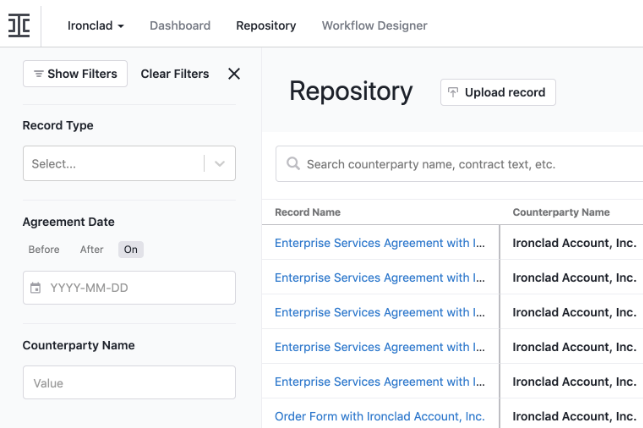
Ironclad's Repository stores executed contracts and the associated metadata, enabling search and reporting across full text and structured metadata.
Setting up a Google Sheet
In this case, we will use Google Sheets as an illustrative example. Create a new Sheet, and go to "Tools" > "Script Editor." This will open the Apps Script editor.
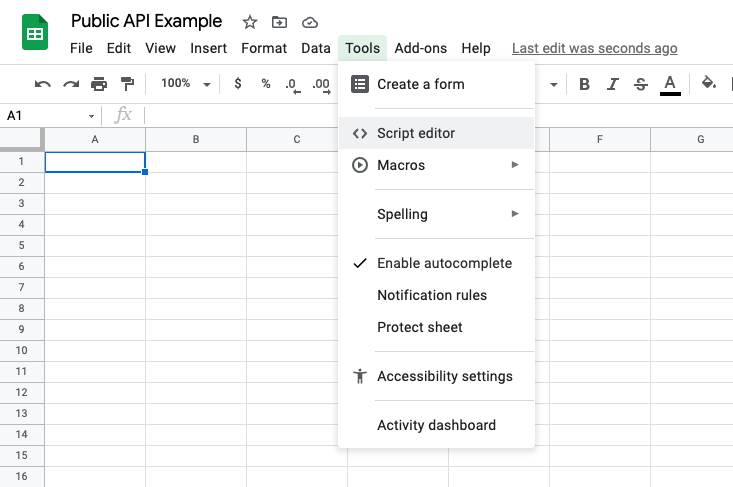
Opening the Script editor in Google Sheets.
Copy in the following code, and replace the API key with your own API key. Then, click "Run" or "Debug."
var apiKey = '[enter your ironclad api key]';
function sync() {
var spreadsheet = SpreadsheetApp.getActiveSpreadsheet();
var sheet = spreadsheet.getSheets()[0];
if (sheet) {
sheet.clear();
}
// setup column headers with record properties
var headers = [];
headers.push('ID');
headers.push('Name');
headers.push('Counterparty Name');
headers.push('Agreement Date');
var data = sheet.getRange(1,1,1,headers.length).setValues([headers]);
// record properties
var records = fetchRecords(records);
for (var j = 0; j < records.length; j++) {
var record = records[j];
var row = [];
row.push(record.id);
row.push(record.name);
row.push(record.properties.counterpartyName && record.properties.counterpartyName.value);
row.push(record.properties.agreementDate && record.properties.agreementDate.value);
sheet.appendRow(row);
}
}
function ICRequest(url) {
var params = {
headers: {
Authorization: 'bearer ' + apiKey
}
};
var resp = UrlFetchApp.fetch('https://demo.ironcladapp.com' + url, params);
if (resp.getResponseCode() >= 400) {
throw new Error('bad response code: ' + resp.getResponseCode() + ' msg: ' + resp.getContentText());
}
return resp;
}
function fetchRecords() {
// Fetch records updated in the last week
var lastUpdated = new Date(Date.now() - 7 * 24 * 60 * 60 * 1000);
var records = [];
var response = {
page: -1,
pageSize: 20,
count: 10,
};
var page = 0;
while ((response.page + 1) * response.pageSize < response.count) {
var rawResponse = ICRequest(`/public/api/v1/records?page=${page}&lastUpdated=${lastUpdated.toISOString()}`);
response = JSON.parse(rawResponse.getContentText());
records = records.concat(response.list);
page++;
}
return records;
}
In the sample code above, the fetchRecords
function calls the Records API endpoint for listing all records, paging through them to collect all record information. It uses the lastUpdated
parameter to only look at Records updated in the past week. This can be helpful for running scheduled jobs, where you only need the integration to consider any updates since the last run.
In the sync
function, we first set the column headers. Then, we iterate through the fetched records, and add them to the spreadsheet as new rows.
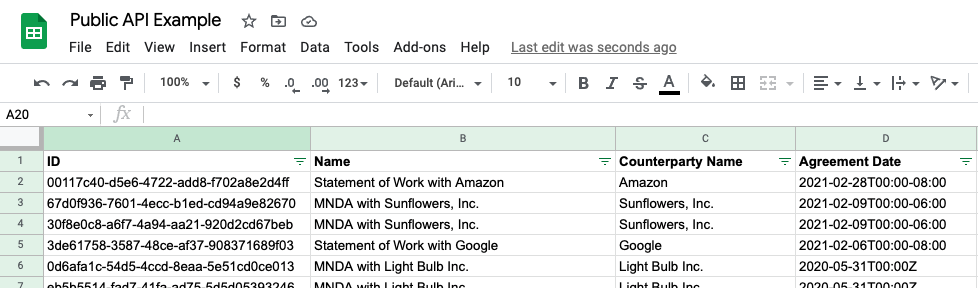
Updated over 1 year ago